코딩항해기
[프로그래머스] PCCE 기출문제 1~9번 본문
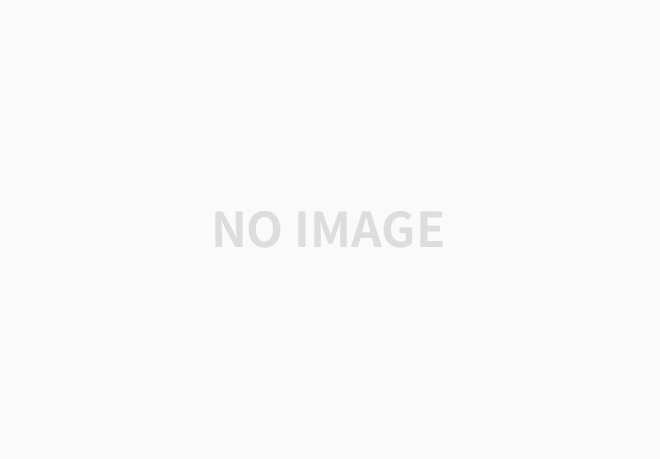
1. 빈칸채우기
import java.util.Scanner;
public class Solution {
public static void main(String[] args) {
String message = "Let's go!";
System.out.println("3\n2\n1");
System.out.println(message);
}
}
2. 디버깅문제
public class Solution {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int angle1 = sc.nextInt();
int angle2 = sc.nextInt();
int sum_angle = (angle1 + angle2) % 360;
System.out.println(sum_angle);
}
}
3. 디버깅문제
public class Solution {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int number = sc.nextInt();
int answer = 0;
while(number>0){
answer += number % 100;
number /= 100;
}
System.out.println(answer);
}
}
4. 빈칸채우기
public class Solution {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String code = sc.next();
String lastFourWords = code.substring(code.length()-4, code.length());
if(lastFourWords.equals(
"_eye"
)){
System.out.println("Ophthalmologyc");
}
else if(
lastFourWords.equals("head")
){
System.out.println("Neurosurgery");
}
else if(
lastFourWords.equals("infl")
){
System.out.println("Orthopedics");
}
else if(lastFourWords.equals("skin"))
{
System.out.println("Dermatology");
}
else
{
System.out.println("direct recommendation");
}
}
}
5. 빈칸채우기
class Solution {
public int[] solution(String[] cpr) {
int[] answer = {0, 0, 0, 0, 0};
String[] basic_order = {"check", "call", "pressure", "respiration", "repeat"};
for(int i=0; i<
cpr.length
; i++){
for(int j=0; j<
basic_order.length
; j++){
if(cpr[i].equals(basic_order[j])){
answer[i] =
j+1
;
break;
}
}
}
return answer;
}
}
6. 디버깅문제
class Solution {
public int solution(int storage, int usage, int[] change) {
int total_usage = 0;
for(int i=0; i<change.length; i++){
usage += usage * change[i] / 100;
total_usage += usage;
if(total_usage > storage){
return i;
}
}
return -1;
}
}
7. 빈칸채우기
class Solution {
public int solution(int seat, String[][] passengers) {
int num_passenger = 0;
for(int i=0; i<passengers.length; i++){
num_passenger += func
4(passengers[i])
;
num_passenger -= func
3(passengers[i])
;
}
int answer = func
1(seat-num_passenger)
;
return answer;
}
8. 디버깅문제
class Solution {
public String solution(String nickname) {
String answer = "";
for(int i=0; i<nickname.length(); i++){
if(nickname.charAt(i) == 'l'){
answer += "I";
}
else if(nickname.charAt(i) == 'w'){
answer += "vv";
}
else if(nickname.charAt(i) == 'W'){
answer += "VV";
}
else if(nickname.charAt(i) == 'O'){
answer += "0";
}
else{
answer += nickname.charAt(i);
}
}
while(answer.length() <= 3){
answer += "o";
}
if(answer.length() > 8){
answer = answer.substring(0, 8);
}
return answer;
}
}
9. 코드작성
(Math를 활용했다면 더 간결한 풀이가 가능했을 것 같다)
class Solution {
public int solution(int[] wallet, int[] bill) {
int answer = 0;
while(true){
if((bill[0] <= wallet[0] && bill[1] <= wallet[1]) || (bill[0] <= wallet[1] && bill[1] <= wallet[0])){
break;
}
if(bill[0]>bill[1]){
bill[0] /= 2;
answer++;
continue;
}
bill[1] /= 2;
answer++;
}
return answer;
}
}
'problem solving > 코딩 테스트' 카테고리의 다른 글
[프로그래머스] JAVA, Python으로 문제풀이 (0) | 2024.11.22 |
---|---|
[코드업] 4833 : 쇠 막대기 (JAVA) (0) | 2024.11.19 |
[프로그래머스/JAVA] [PCCE 기출문제] 1번~8번 (0) | 2024.07.06 |
[프로그래머스/JAVA] 가장 많이 받은 선물 (1) | 2024.07.05 |
[프로그래머스/JAVA] 나이 출력 (0) | 2024.07.01 |